A Philosophy of Software Design: Building Better Code from the Ground Up
In the world of software development, writing code is just the beginning. The real art—and challenge—lies in designing software that’s not only functional but flexible, maintainable, and scalable. That’s where the philosophy of software design steps in.
Whether you’re a junior developer or a seasoned architect, embracing a sound design philosophy can transform the way you write and think about code. Let’s dive into the core principles, common pitfalls, and best practices that shape a thoughtful approach to software design.
What Is a Philosophy of Software Design?
At its core, a philosophy of software design is a set of guiding principles that help developers create systems that stand the test of time. It’s not about specific technologies or languages—it’s about how to think when crafting software.
Key Goals of Good Software Design
- Reduce complexity
- Improve readability
- Enable reuse and extensibility
- Minimize technical debt
This philosophy helps developers move beyond quick fixes and short-term gains toward long-term stability and clarity.
The Complexity Trap: The Enemy of Good Design
One of the biggest threats to good software is unnecessary complexity. Codebases often become harder to understand and modify as they grow—not because they’re doing more, but because of how they’re structured.
Symptoms of High Complexity
- Spaghetti code with unclear flow
- Repeated logic across multiple files
- Difficult-to-change features
- Overuse of abstractions without clear benefit
Great design isn’t about adding more layers—it’s about removing unnecessary ones.
Core Principles of Thoughtful Software Design
Let’s explore some of the fundamental principles that drive clean, effective, and future-proof software design.
1. Design for Change
Software is never truly finished. Requirements shift, new features emerge, and users evolve. A good design anticipates this reality.
How to apply:
- Favor modular architecture so individual parts can change independently.
- Use clear interfaces that decouple dependencies.
- Write code that’s easy to read and revisit months—or years—later.
2. Minimize Dependencies
Every dependency is a liability. The more one component relies on another, the harder it becomes to change either.
Best practices:
- Adhere to the Single Responsibility Principle (SRP).
- Isolate external systems (like APIs or databases) behind clean wrappers.
- Leverage dependency injection to reduce tight coupling.
3. Favor Simplicity Over Cleverness
Clever code may impress at first glance, but simple code endures. It’s easier to test, debug, and extend.
Ask yourself:
- Would a new developer understand this logic?
- Is this the simplest solution that gets the job done?
- Can this function be broken into smaller, clearer pieces?
The Role of Naming, Comments, and Code Structure
Design isn’t just about architecture—it’s also about how you write the day-to-day code.
Good Naming: A Hidden Superpower
Clear, descriptive names act like documentation baked into your code.
- Use verbs for functions (e.g.,
fetchUserData()
) - Use nouns for variables and classes (e.g.,
userList
,InvoiceManager
) - Avoid abbreviations unless universally understood
Write Comments with Purpose
Comments should explain why, not what. Good code speaks for itself, but great code still benefits from context.
- Avoid redundant comments
- Use them to explain design decisions, trade-offs, or future concerns
Refactoring: The Continuous Path to Better Design
Refactoring is not just cleanup—it’s a core part of the design process. As code evolves, so must its structure.
Effective refactoring habits:
- Do it regularly, not just during “cleanup sprints”
- Use tests to ensure changes don’t break functionality
- Look for code smells: duplicated code, long methods, or large classes
Real-World Examples of Design Done Right
Let’s look at how these principles apply in actual software projects.
Example 1: Microservices Done Well
In a well-designed microservice architecture:
- Each service is focused on a single business function
- APIs are well-documented and versioned
- Failure in one service doesn’t bring down the entire system
Example 2: Scalable Frontend Architecture
In modern frontend frameworks like React or Vue:
- Components are small, focused, and reusable
- State is managed clearly using tools like Redux or Vuex
- Styling is isolated to avoid conflicts and bloat
These architectures succeed not because of tools, but because of how the teams approach design decisions.
Conclusion: Design with Intention, Code with Confidence
Software design isn’t just for architects—it’s for everyone who writes code. By embracing a thoughtful design philosophy, you:
- Write cleaner, simpler code
- Spend less time debugging and more time building
- Build systems that scale with your users and your team
Start today: the next time you sit down to write a feature or fix a bug, ask yourself:
“Is this the simplest, clearest way to solve this problem?”
That small shift in mindset can lead to big, lasting improvements.
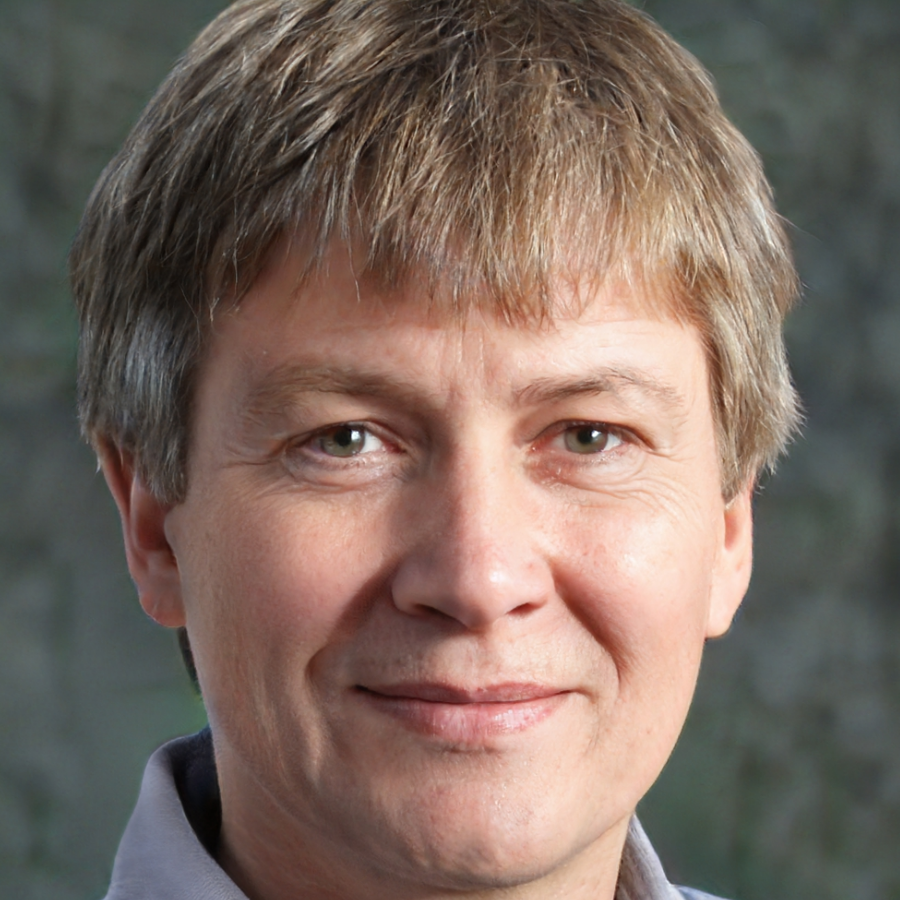
Zain Jacobs is a writer for TheNetUse, providing in-depth reviews, tips, and insights on the latest software apps to help users maximize their digital experience.